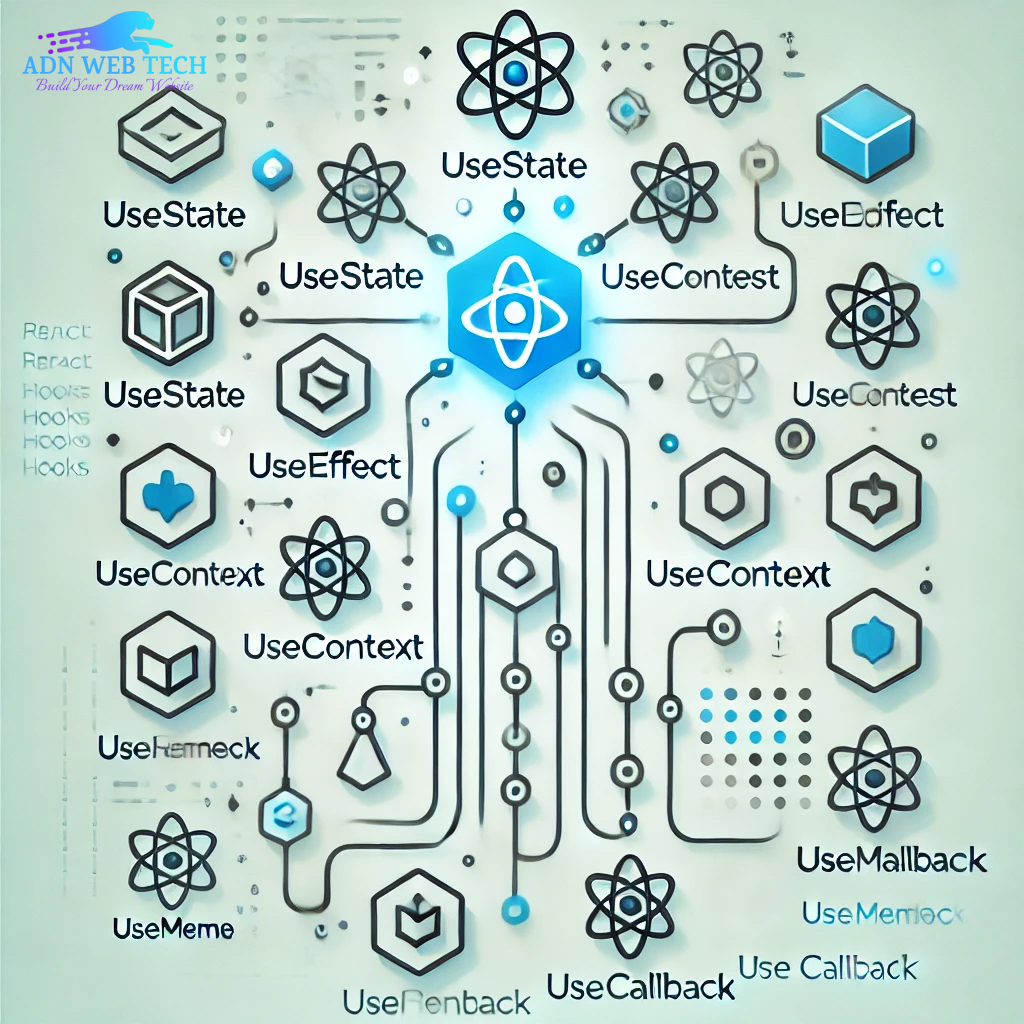
Mastering React Hooks: A Complete Guide for Modern React Development
What Are React Hooks?
React Hooks are JavaScript functions that enable developers to use React features (like state, context, and lifecycle methods) in functional components. They offer a simpler, more declarative way to manage component state and side effects compared to class components. Hooks not only make code more readable but also improve the reusability of logic across components.
Why Were React Hooks Introduced?
React Hooks were introduced to solve several limitations and complexities of class components:
- Simplified Component Logic: Managing state and lifecycle methods in class components can be tricky and error-prone. React Hooks eliminate that complexity.
- Enhanced Code Reusability: Hooks enable better reuse of component logic without relying on higher-order components (HOCs) or render props.
- Improved Readability: Hooks streamline the code, making it more readable and easier to maintain.
- Incremental Adoption: You can use Hooks alongside class components, allowing a gradual migration toward functional components.
Commonly Used React Hooks
Let’s explore the key React Hooks and how they help you build modern React applications:
1. useState
: Managing State in Functional Components
The useState
hook allows you to manage state in functional components, a feature previously only available in class components.
Example:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0); // Initialize state
return (
Current Count: {count}
);
} export default Counter;
Explanation:
useState(0)
initializes thecount
state to 0.setCount(count + 1)
updates the state when the button is clicked.
2. useEffect
: Handling Side Effects
useEffect
is used for side effects like data fetching, subscriptions, or manually changing the DOM.
Example:
import React, { useState, useEffect } from 'react';
function Timer() {
const [time, setTime] = useState(0);
useEffect(() => { const interval = setInterval(() => setTime(t => t + 1), 1000);
return () => clearInterval(interval); // Cleanup on unmount
}, []); // Runs once on mount
return Elapsed Time: {time}s
;
} export default Timer;
Explanation:
- The
useEffect
hook starts an interval that updates the time every second. - The cleanup function (
clearInterval
) ensures that the timer is cleared when the component unmounts.
3. useContext
: Accessing Context Values
useContext
allows functional components to access context values, eliminating the need for prop drilling.
Example:
import React, { useContext, createContext } from 'react';
const ThemeContext = createContext('light');
function ThemedButton() {
const theme = useContext(ThemeContext);
return ;
} function App() {
return ( );
} export default App;
Explanation:
useContext(ThemeContext)
fetches the current value ofThemeContext
, allowingThemedButton
to apply the correct theme.
4. useReducer
: Managing Complex State
When you need to manage more complex state logic, useReducer
is a great alternative to useState
.
Example:
import React, { useContext, createContext } from 'react';
const ThemeContext = createContext('light');
function ThemedButton() {
const theme = useContext(ThemeContext);
return ;
}
function App() {
return (
);
} export default App;
Explanation:
useReducer
manages state transitions based on dispatched actions, making it useful for handling more complex logic.
5. useRef
: Persisting Values
useRef
allows you to persist values across renders without triggering a re-render.
Example:
import React, { useRef } from 'react';
function FocusInput() {
const inputRef = useRef();
const focus = () => inputRef.current.focus();
return ( );
} export default FocusInput;
Explanation:
useRef
is used to reference the DOM element and focus it when the button is clicked.
React Hooks have simplified component logic and state management, enabling more reusable and readable code. By using hooks like useState
, useEffect
, useReducer
, and others, you can build modern, performant React applications. Mastering these hooks is essential for React developers looking to unlock the full potential of React’s capabilities.
Start incorporating React Hooks into your projects today, and elevate your React development skills to the next level!